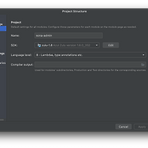
build.gradle 설정 sourceCompatibility = '1.8' targetCompatibility = '1.8' plugins { id 'java' } java { sourceCompatibility = '1.8' targetCompatibility = '1.8' } sourceCompatibility 는 개발할 때 작성하는 자바 소스 코드의 버전이고, targetCompatibility 는 소스를 컴파일해서 생성되는 class 파일의 버전으로 프로그램이 수행될 수 있는 최소 자바 버전이 됩니다. 자바 11로 개발하지만 자바 8에 호환가능 하도록 배포를 하려면 source는 11, targert은 1.8로 설정을 하면 됩니다. IntelliJ 설정 File > Project Structu..
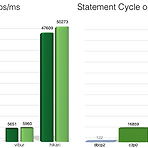
Spring Boot JPA(2.x.x 기준)에서 사용 가능한 Connection Pool은 아래의 3가지가 있습니다. HikariCP Tomcat pooling Datasource Commons DBCP2 Spring Boot 1.x.x 에서는 Tomcat을 Default로 사용하였으나 Spring Boot 2.0.0 에서부터 default JDBC connection pool(이하 'CP')을 Tomcat에서 HikariCP로 변경하였습니다. 그래서 spring-boot-starter-jdbc, spring-boot-starter-data-jpa를 사용하면, HikariCP(가장 높음) -> Tomcat pooling -> Commons DBCP2 의 우선 순위로 CP가 적용 됩니다. 위의 우선순위를..
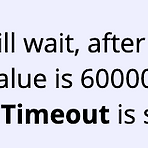
API 요청의 처리가 오래 걸리게 되면, java.net.SocketTimeoutException 가 발생합니다. tomcat 문서를 보면, defualt 값은 1분으로 되어 있습니다.( tomcat 8.5 버전 기준 ) Spring의 property 파일( application.yaml )의 timeout 시간을 늘려주면 됩니다. -1을 설정하면 timeout이 무한대가 되어 timeout이 발생하지 않습니다. 그렇지 않으면 ms 단위로 원하는 시간을 입력해주면 됩니다. server: tomcat: connection-timeout: -1
Jackson objectmapper는 Java 오브젝트와 JSON 간에 직렬화, 역직렬화를 해주는 역할을 합니다. Jackson 사용을 위해 의존성을 추가합니다. databind는 jackson-annotations, jackson-core 의존성도 포함되어 있습니다. com.fasterxml.jackson.core jackson-databind 2.13.2.2 Cat 클래스를 이용하여 직렬화, 역직렬화를 해보겠습니다. @Getter @Setter @NoArgsConstructor public class Cat { private String name; public Cat(String name) { this.name = name; } } Object to Json ObjectMapper objectMap..
Jackson으로 객체를 직렬화 할 때, 아래와 같이 에러가 발생할 때가 있습니다. 직렬화를 할 때, 객체의 getter를 사용하여 변환을 하는데 이 때 에러가 발생하면 아래와 같은 예외가 발생합니다. Exception in thread "main" com.fasterxml.jackson.databind.exc.InvalidDefinitionException: No serializer found for class com.example.springapp.animal.Cat and no properties discovered to create BeanSerializer (to avoid exception, disable SerializationFeature.FAIL_ON_EMPTY_BEANS) 코드를 보면 ..
Spring에서 HTTP 요청을 하기 위해 RestTemplate를 많이 사용합니다. ( Spring 5.0부터 RestTemplate대신 WebClient을 사용을 추천하므로 legacy 프로젝트를 하시는 것이 아니라면 WebClient로 개발하시는 것을 추천드립니다. RestTemplate는 5.0부터 유지보수만 이루어집니다. 관련 링크 ) RestTemplate의 exchange, getForEntity, postForEntity 등의 API를 이용하여 응답을 ResponseEntity로 한 번에 메모리에 받아옵니다. 일반적인 경우에는 문제가 되지 않지만 응답의 크기가 수백MB이상이 되면 GC가 발생하게 됩니다. 저도 프로젝트를 진행하는 와중에, GC가 발생하여 확인해보니 응답 사이즈가 600MB ..
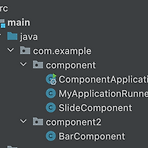
목차 ComponentScan 이란? @ComponentScan은 스프링부트에서 제공하는 어노테이션으로써, 스프링 빈으로 만들기 위한 컴포넌트를 스캔할 곳을 설정하는 역할을 합니다. 스프링에서는 여러 빈들을 관리하면서 의존성을 주입해 주는데 이때 전체 코드에서 컴포넌트를 찾아 빈을 생성하는 것이 아니라 범위를 지정하여 그 범위 안에서 어노테이션 된 클래스들을 찾아 빈을 생성 및 관리합니다. 빈으로 등록하기 위해 스캔하는 어노테이션은 @Component, @Repository, @Service, @Controller, @Configuration 가 있습니다. com.example.component.SlideComponent와 com.example.component2.BarComponent가 있는데 둘은 서..
resources > res.txt 파일을 읽기 위해서 아래와 같이 ResourceLoader를 이용하여 classpath에 있는 파일을 읽을 수 있습니다. package com.example.logbacksample; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.ApplicationArguments; import org.springframework.boot.ApplicationRunner; import org.springframework.core.io.ResourceLoader; import org.springframe..
property에 있는 값을 @Value를 사용하여 멤버 변수에 값을 Injection하여 자주 사용을 합니다. 이때 static 멤버에 Injection을 하려고 하면 되지가 않습니다. String 변수를 예로 들면, null로 되어있습니다. 이유는 Spring에서 @Value 가 static field에 대해 지원하지 않기 때문입니다. public class Inject { @Value("${file.path}") private static String PATH; // null } static 변수에 Injection을 하려면, 아래와 같이 setter injection으로 값을 할당할 수 있습니다. public class Inject { private static String PATH; @Value..
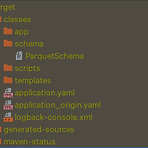
ResourceLoader는 Spring Boot에서 resources 폴더 아래에 있는 리소스 파일을 읽어오기 위해 사용하는 클래스 입니다. 아래와 같이 classpath:로 경로를 입력하면 해당 경로에 있는 리소스를 읽어오고 Resource 객체로 full path까지 가져올 수 있습니다. ResourceLoader resourceLoader; Resource resource = resourceLoader.getResource("classpath:" + schemaFilePath); resource.getURI().getPath(); 여기서 classpath: 는 target의 classes를 root로 하는 경로 입니다. chemaFilePath는 schema/ParquetSchema 값을 넣어주면..
- Total
- Today
- Yesterday
- Container
- mac
- Git
- spring boot
- Log
- plugin
- SpringBoot
- JPA
- Postman
- tomcat
- gradle
- Spark
- apm
- scala
- spring
- Filter
- elasticsearch
- Linux
- AWS
- Index
- intellij
- maven
- Size
- logstash
- Kibana
- JSON
- docker
- install
- error
- Java
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |